Estimated Reading Time: 4 min
Here are some essential WordPress PHP functions and tricks that can help you customize and improve your WordPress website:
1. Display the Site URL Dynamically
echo get_site_url();
This function retrieves the URL of your WordPress site.
2. Get the Theme Directory URL
echo get_template_directory_uri();
Useful when you want to include assets (CSS, JS, images) from your theme folder.
3. Add Custom Styles and Scripts
function custom_theme_assets() {
wp_enqueue_style('custom-style', get_stylesheet_uri());
wp_enqueue_script('custom-js', get_template_directory_uri() . '/js/custom.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'custom_theme_assets');
This ensures proper loading of styles and scripts in WordPress.
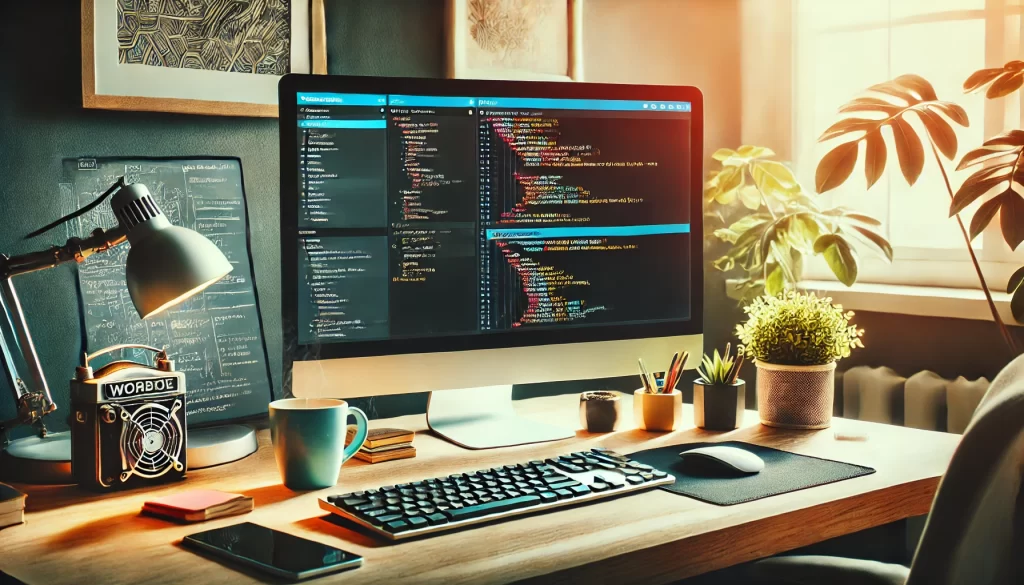
4. Create a Custom Excerpt Length
function custom_excerpt_length($length) {
return 20; // Change to desired word count
}
add_filter('excerpt_length', 'custom_excerpt_length');
This modifies the number of words displayed in excerpts.
5. Redirect Users After Login
function custom_login_redirect($redirect_to, $request, $user) {
if (isset($user->roles) && is_array($user->roles)) {
if (in_array('administrator', $user->roles)) {
return admin_url(); // Redirect admin to dashboard
} else {
return home_url(); // Redirect other users to homepage
}
}
return $redirect_to;
}
add_filter('login_redirect', 'custom_login_redirect', 10, 3);
This function redirects users based on their roles after login.
6. Disable WordPress Admin Bar for Non-Admins
function disable_admin_bar() {
if (!current_user_can('administrator')) {
show_admin_bar(false);
}
}
add_action('after_setup_theme', 'disable_admin_bar');
Removes the admin bar for all users except administrators.
7. Add Custom Image Sizes
function custom_image_sizes() {
add_image_size('custom-size', 800, 600, true);
}
add_action('after_setup_theme', 'custom_image_sizes');
You can then use:
the_post_thumbnail('custom-size');
to display images in this custom size.
8. Remove WordPress Version for Security
function remove_wp_version() {
return '';
}
add_filter('the_generator', 'remove_wp_version');
This hides the WordPress version from HTML source code to reduce security risks.
9. Create a Custom Widget Area
function custom_widget_area() {
register_sidebar(array(
'name' => 'Custom Sidebar',
'id' => 'custom-sidebar',
'before_widget' => '<div class="widget">',
'after_widget' => '</div>',
'before_title' => '<h3>',
'after_title' => '</h3>',
));
}
add_action('widgets_init', 'custom_widget_area');
This allows users to add widgets to a new sidebar in the WordPress admin.
10. Display the Last Updated Date of a Post
function last_updated_date($content) {
if (is_single() && get_the_modified_time() != get_the_time()) {
$updated = '<p>Last updated on: ' . get_the_modified_time('F j, Y') . '</p>';
return $updated . $content;
}
return $content;
}
add_filter('the_content', 'last_updated_date');
This will show the last updated date before the post content.
11. Add Custom Menu Support
function custom_menus() {
register_nav_menus(array(
'header-menu' => __('Header Menu'),
'footer-menu' => __('Footer Menu'),
));
}
add_action('after_setup_theme', 'custom_menus');
You can then call the menu in a theme file using:
wp_nav_menu(array('theme_location' => 'header-menu'));
12. Redirect 404 Errors to Home Page
function redirect_404_to_home() {
if (is_404()) {
wp_redirect(home_url());
exit();
}
}
add_action('template_redirect', 'redirect_404_to_home');
This function redirects all 404 errors to the homepage.
13. Remove WordPress Emoji Script for Performance
function disable_wp_emoji() {
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('wp_print_styles', 'print_emoji_styles');
}
add_action('init', 'disable_wp_emoji');
This helps speed up your WordPress site by disabling emoji scripts.
14. Limit WordPress Post Revisions
define('WP_POST_REVISIONS', 5);
Add this to wp-config.php
to limit the number of saved revisions for each post.
15. Enable SVG Uploads
function allow_svg_upload($mimes) {
$mimes['svg'] = 'image/svg+xml';
return $mimes;
}
add_filter('upload_mimes', 'allow_svg_upload');
This allows SVG file uploads in WordPress.
These functions and tricks will help you enhance, secure, and optimize your WordPress website. Let me know if you need something more specific! 🚀