Estimated Reading Time: 2 min
Overloading the functions.php
file can lead to performance issues, code organization problems, and maintenance challenges. Here’s how to avoid overloading functions.php
effectively:
Why Avoid Overloading functions.php
?
- Performance: Too much code in
functions.php
can slow down your site. - Maintainability: A cluttered
functions.php
becomes hard to read, debug, and update. - Portability: Code in
functions.php
is tied to a specific theme. Switching themes breaks the functionality.
Best Practices to Avoid Overloading functions.php
1. Use Custom Plugins for Functionality
- For functionality unrelated to the theme (e.g., custom post types, taxonomies, or shortcodes), create a custom plugin instead of adding code to
functions.php
. - Place this file in the
wp-content/plugins
directory and activate it via the WordPress dashboard. - Example:
<?php /* Plugin Name: Custom Post Type Plugin Description: A plugin for custom post types. */ add_action('init', function() { register_post_type('custom_post', [ 'public' => true, 'label' => 'Custom Posts' ]); });
2. Organize Code into Include Files
- Break down your code into smaller, topic-specific files and include them in
functions.php
. Example:
Folder Structure: theme/ ├── functions/ │ ├── custom-post-types.php │ ├── enqueue-scripts.php │ ├── theme-setup.php └── functions.php
functions.php: require get_template_directory() . '/functions/theme-setup.php'; require get_template_directory() . '/functions/enqueue-scripts.php'; require get_template_directory() . '/functions/custom-post-types.php';
3. Use Classes or Namespaces
- Encapsulate your functionality in classes or namespaces for better code organization and to avoid conflicts. Example:
namespace MyTheme; class ThemeSetup { public static function init() { add_action('after_setup_theme', [self::class, 'setup']); } public static function setup() { add_theme_support('title-tag'); add_theme_support('post-thumbnails'); } } ThemeSetup::init();
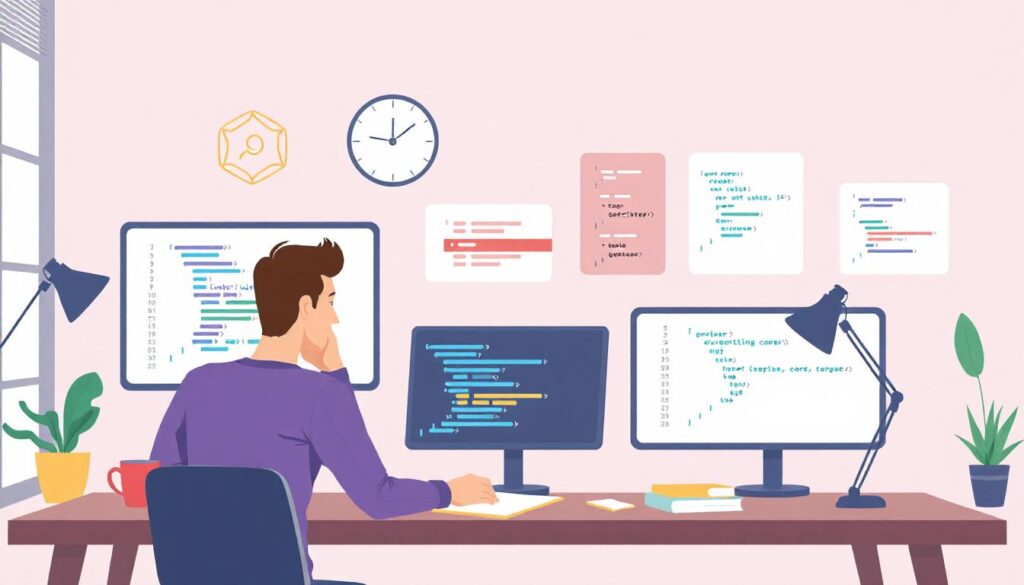
4. Limit Code in functions.php
- Only include essential theme-specific setup functions, like registering menus, widget areas, or theme support features. Example:
add_action('after_setup_theme', function() { add_theme_support('custom-logo'); register_nav_menu('primary', 'Primary Menu'); });
5. Use mu-plugins
for Must-Have Features
- Place critical features in the
wp-content/mu-plugins
directory. These plugins load automatically and aren’t tied to the theme.
Key Takeaways
- Reserve
functions.php
for theme-specific features only. - Use plugins for site-wide functionality.
- Organize large codebases into separate files or use object-oriented programming.