Estimated Reading Time: 7 min
Want to keep visitors engaged and boost your site’s SEO? A related posts section helps users discover more content they’ll love while increasing page views and reducing bounce rates. In this guide, we’ll show you how to create a custom related posts section for your WordPress site using PHP, CSS, and JavaScript, no plugins required! Follow along for a step-by-step tutorial to enhance your blog’s user experience. 🚀 Here are several methods you can use:
1. Using a WordPress Plugin (Easy Method)
There are several plugins that can automatically generate related posts based on categories, tags, or content analysis.
Recommended Plugins:
- Yet Another Related Posts Plugin (YARPP) – Uses a customizable algorithm to find related posts.
- Contextual Related Posts – Uses full-text scanning for better post matching.
- Related Posts for WordPress – A lightweight option for fast performance.
How to Install & Use:
- Go to your WordPress dashboard.
- Navigate to Plugins > Add New.
- Search for one of the plugins above and install it.
- Activate the plugin and configure settings under Settings > Related Posts.
- Adjust how related posts are displayed (e.g., by category, tags, or content).
2. Using Yoast SEO’s Related Links (If You Have Yoast Installed)
Yoast SEO Premium has an internal linking tool that suggests related posts while editing content. You can manually add these suggestions within your posts.
How to Use:
- When editing a post, check the Yoast SEO sidebar.
- Under “Internal Linking Suggestions,” select and link relevant posts.
- Add them manually in a “Related Posts” section.
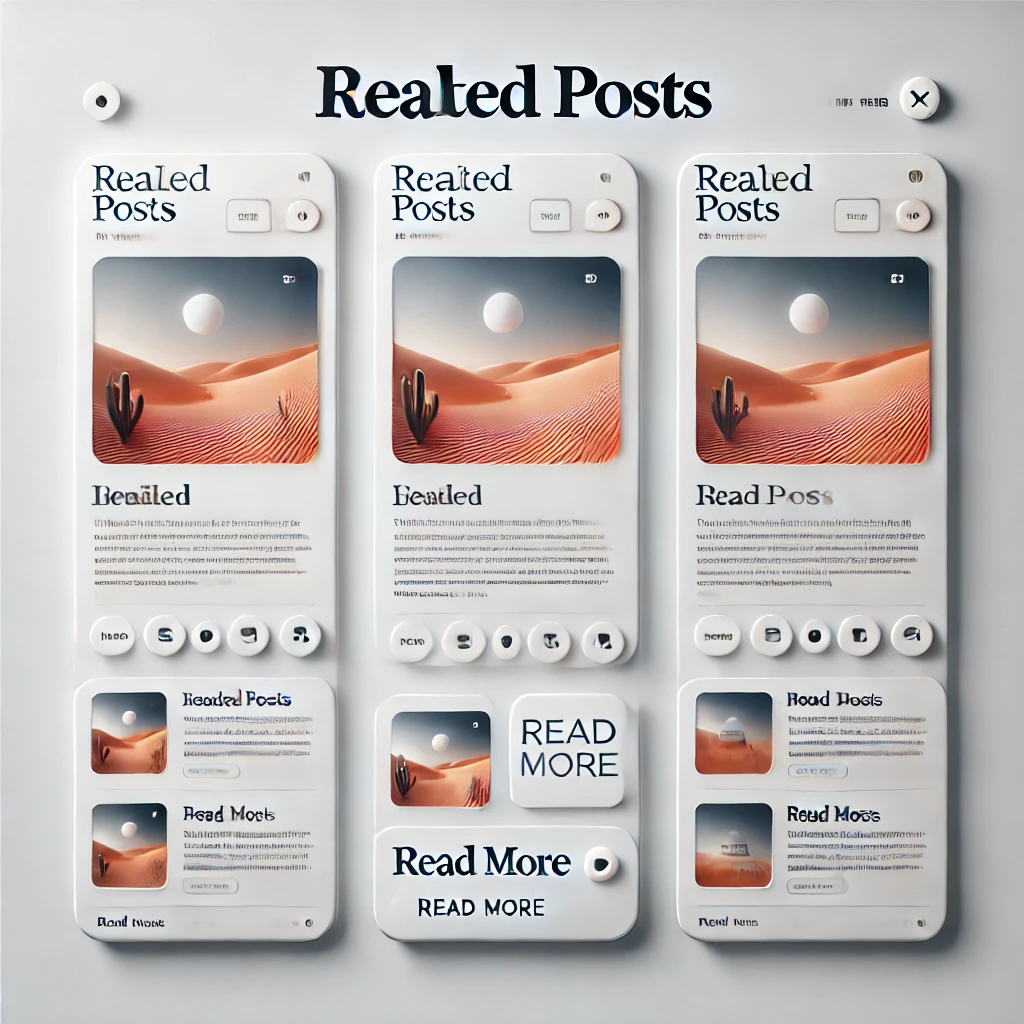
3. Manually Adding Related Posts (Basic Code Method)
If you prefer a lightweight approach, you can manually add a related posts section using PHP.
Steps to Add Code to Your Theme:
- Go to Appearance > Theme Editor.
- Open
single.php
orcontent-single.php
(depending on your theme). - Add the following code before the comments section:
<?php
$categories = get_the_category();
if ($categories) {
$category_ids = array();
foreach ($categories as $category) {
$category_ids[] = $category->term_id;
}
$query_args = array(
'category__in' => $category_ids,
'post__not_in' => array(get_the_ID()),
'posts_per_page' => 4, // Adjust number of posts
'orderby' => 'rand' // Show random related posts
);
$related_posts = new WP_Query($query_args);
if ($related_posts->have_posts()) {
echo '<h3>Related Posts</h3><ul>';
while ($related_posts->have_posts()) {
$related_posts->the_post(); ?>
<li>
<a href="<?php the_permalink(); ?>"><?php the_title(); ?></a>
</li>
<?php }
echo '</ul>';
}
wp_reset_postdata();
}
?>
What This Does:
- Finds posts in the same category.
- Excludes the current post.
- Displays 4 random related posts.
4. Using a Block Editor or Elementor (No Plugin, No Code)
If you’re using Gutenberg or Elementor, you can manually create a related posts section.
Gutenberg (Block Editor)
- Edit a post and insert a “Latest Posts” block.
- Set it to display posts from the same category.
- Customize layout (list, grid, etc.).
- Add a title like “Related Posts.”
Elementor
- Drag and drop the “Posts” widget into your post.
- Filter posts by category or tags.
- Customize display settings.
5. Using the_content
Hook (Best for Inserting After Post Content)
This will automatically append related posts at the end of each blog post.
Steps to Implement:
- Open your theme’s
functions.php
file. - Add this code:
function besmart_related_posts($content) {
if (is_single() && in_the_loop() && is_main_query()) {
ob_start(); // Start output buffering
?>
<div class="besmart-related-posts">
<h3>Related Posts</h3>
<ul>
<?php
$categories = get_the_category();
if ($categories) {
$category_ids = array_map(function($cat) { return $cat->term_id; }, $categories);
$query_args = array(
'category__in' => $category_ids,
'post__not_in' => array(get_the_ID()),
'posts_per_page' => 4, // Adjust as needed
'orderby' => 'rand',
);
$related_posts = new WP_Query($query_args);
if ($related_posts->have_posts()) {
while ($related_posts->have_posts()) {
$related_posts->the_post();
?>
<li>
<a href="<?php the_permalink(); ?>"><?php the_title(); ?></a>
</li>
<?php
}
wp_reset_postdata();
} else {
echo '<li>No related posts found.</li>';
}
}
?>
</ul>
</div>
<?php
$related_posts_content = ob_get_clean(); // Get buffered content
$content .= $related_posts_content; // Append to post content
}
return $content;
}
add_filter('the_content', 'besmart_related_posts');
What This Does:
✅ Automatically adds Related Posts below each blog post.
✅ Shows random posts from the same category.
✅ Lightweight – no plugin required.
6. Using wp_footer
Hook (If You Want It in the Footer)
This method displays related posts at the bottom of the page.
Code for functions.php
:
function besmart_related_posts_footer() {
if (is_single()) {
?>
<div class="besmart-related-posts-footer">
<h3>Related Posts</h3>
<ul>
<?php
$categories = get_the_category();
if ($categories) {
$category_ids = array_map(fn($cat) => $cat->term_id, $categories);
$query_args = array(
'category__in' => $category_ids,
'post__not_in' => array(get_the_ID()),
'posts_per_page' => 4,
'orderby' => 'rand',
);
$related_posts = new WP_Query($query_args);
if ($related_posts->have_posts()) {
while ($related_posts->have_posts()) {
$related_posts->the_post();
?>
<li><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></li>
<?php
}
wp_reset_postdata();
} else {
echo '<li>No related posts available.</li>';
}
}
?>
</ul>
</div>
<?php
}
}
add_action('wp_footer', 'besmart_related_posts_footer');
Best Use Case:
✅ Displays Related Posts at the bottom of the entire page.
✅ Good if you have a sticky footer layout.
7. Using a Custom Hook (For Theme-Specific Placement)
If your theme has an after_post
hook, you can add related posts right after the post body.
Code for functions.php
:
function besmart_related_posts_after_post() {
if (is_single()) {
echo '<div class="besmart-related-posts">';
echo '<h3>Related Posts</h3><ul>';
$categories = get_the_category();
if ($categories) {
$category_ids = array_map(fn($cat) => $cat->term_id, $categories);
$query_args = array(
'category__in' => $category_ids,
'post__not_in' => array(get_the_ID()),
'posts_per_page' => 4,
'orderby' => 'rand',
);
$related_posts = new WP_Query($query_args);
if ($related_posts->have_posts()) {
while ($related_posts->have_posts()) {
$related_posts->the_post();
echo '<li><a href="' . get_permalink() . '">' . get_the_title() . '</a></li>';
}
wp_reset_postdata();
} else {
echo '<li>No related posts found.</li>';
}
}
echo '</ul></div>';
}
}
add_action('after_post', 'besmart_related_posts_after_post');
👉 Note: Works only if your theme has an after_post
hook. If it doesn’t work, use the_content
.
BONUS. Custom Styling
To make the related posts section look nice, add this to your style.css
:
.besmart-related-posts, .besmart-related-posts-footer {
margin-top: 30px;
padding: 20px;
background: #f1f1f1;
border-left: 5px solid #2c3e50;
border-radius: 5px;
}
.besmart-related-posts h3, .besmart-related-posts-footer h3 {
font-size: 18px;
margin-bottom: 10px;
}
.besmart-related-posts ul, .besmart-related-posts-footer ul {
list-style: none;
padding-left: 0;
}
.besmart-related-posts li, .besmart-related-posts-footer li {
margin: 5px 0;
}
.besmart-related-posts a, .besmart-related-posts-footer a {
text-decoration: none;
color: #2c3e50;
}
.besmart-related-posts a:hover, .besmart-related-posts-footer a:hover {
text-decoration: underline;
}
Which Hook Should You Use?
- Best for below post content →
the_content
- Best for footer placement →
wp_footer
- Best if your theme has an after_post hook →
after_post
Which Method Should You Choose?
- Beginners: Use a plugin like YARPP or Contextual Related Posts.
- SEO-focused users (Yoast users): Manually insert internal links suggested by Yoast.
- Minimalists (No Plugin, No Code): Use the Gutenberg “Latest Posts” block.
- Developers & Advanced Users: Add PHP code to the theme for a lightweight approach.
Let me know if you need help implementing any of these! 🚀