Estimated Reading Time: 3 min
To create a “Trump Ticker” price widget for a WordPress site, pulling live price data from Binance, you would need to use the Binance API to fetch the data and display it on your WordPress page. Here is a simple step-by-step guide on how to do that.
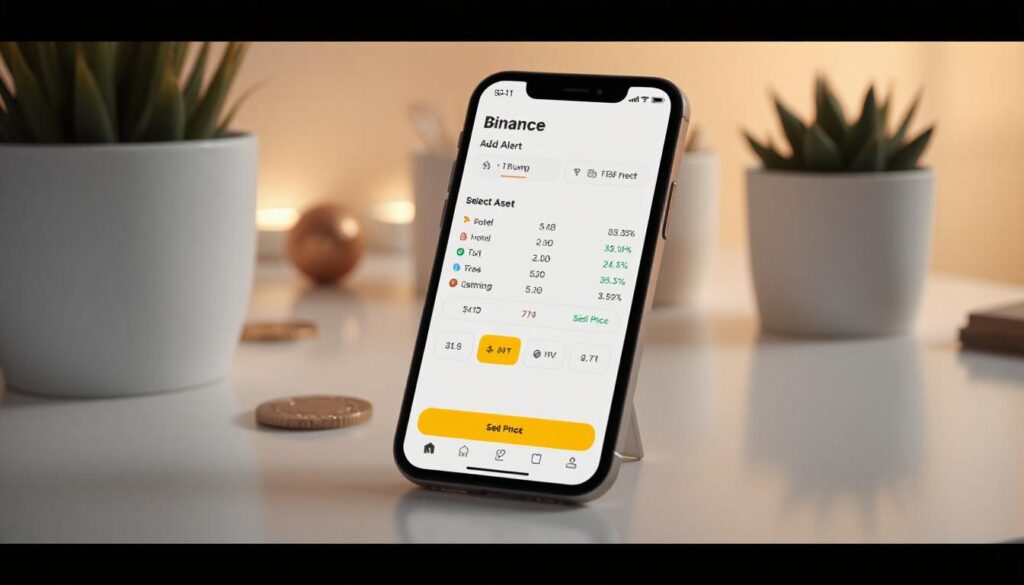
Steps to Implement the “Trump Ticker Price” Widget:
- Create a Binance API Key:
- Go to your Binance account.
- Navigate to the API Management section under the user profile.
- Create a new API key (you will get an API key and secret key).
- Use the API key for fetching the data.
- Install a Custom Plugin or Use Existing Ones: You can either write a custom plugin to fetch and display the price or use an existing plugin like “WP Code” (formerly “Insert Headers and Footers”).
- Write Custom Code: Below is a simple PHP code snippet you can add to your custom plugin or the functions.php file of your WordPress theme.
- PHP Code to Fetch Data from Binance API: You can use PHP to call the Binance API and display the price. Here’s a basic example:
<?php
function get_binance_price() {
$url = "https://api.binance.com/api/v3/ticker/price?symbol=TRUMPUSDT"; // Replace "TRUMPUSDT" with the correct symbol if needed
$response = wp_remote_get($url);
if (is_wp_error($response)) {
return 'Error fetching data';
}
$body = wp_remote_retrieve_body($response);
$data = json_decode($body, true);
if (isset($data['price'])) {
return 'Trump Token Price: $' . $data['price'];
} else {
return 'Price data not available';
}
}
function display_trump_ticker() {
echo '<div class="trump-ticker-widget">';
echo '<h2>' . get_binance_price() . '</h2>';
echo '</div>';
}
add_shortcode('trump_ticker', 'display_trump_ticker');
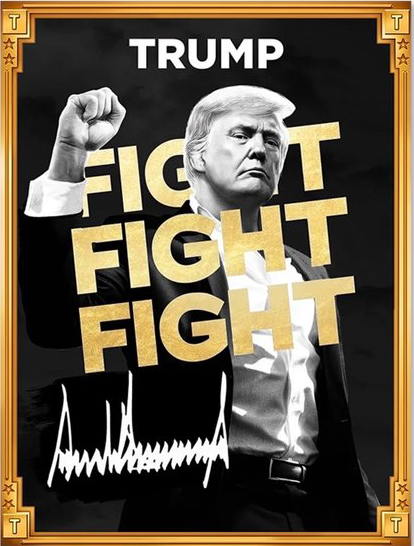
How to Use:
- Step 1: Add the code above to your theme’s
functions.php
or a custom plugin. - Step 2: Use the shortcode
[trump_ticker]
anywhere in your posts or pages where you want to display the price.
Explanation of the Code:
- The function
get_binance_price
uses the Binance API endpoint to get the latest price of the “TRUMPUSDT” pair. You can replace “TRUMPUSDT” with the correct pair if needed. - The
wp_remote_get
function is used to fetch the price from the API. - The
display_trump_ticker
function echoes the price inside a styled div. - The shortcode
[trump_ticker]
allows you to insert the ticker anywhere in your content.
Optional Enhancements:
- Styling: Add custom CSS to style the ticker widget as per your site’s design.
- Error Handling: Implement more robust error handling to catch API issues.
- JavaScript: You could also refresh the price periodically using JavaScript and AJAX to keep the data up to date.
Let me know if you’d like additional details or adjustments to this code!