Estimated Reading Time: 3 min
Eliminating render-blocking scripts is crucial for improving page speed and SEO. Here’s a step-by-step guide to do it effectively:
1. Identify Render-Blocking Scripts
Use tools like:
- Google PageSpeed Insights (https://pagespeed.web.dev/)
- GTmetrix (https://gtmetrix.com/)
- Chrome DevTools (Lighthouse & Performance tab)
Look for scripts flagged as render-blocking, typically <script>
tags in the <head>
that block page rendering.
2. Defer or Async JavaScript Loading
Modify your scripts to prevent them from blocking the rendering of the page.
Use defer
for non-essential scripts
<script src="script.js" defer></script>
- Execution order is maintained (scripts load in order)
- Scripts load asynchronously but execute after the DOM is fully parsed.
Use async
for independent scripts
<script src="script.js" async></script>
- Loads asynchronously and executes immediately when downloaded.
- Can cause race conditions if dependencies exist.
✅ Best practice: Use defer
for most scripts, async
for analytics and ads.
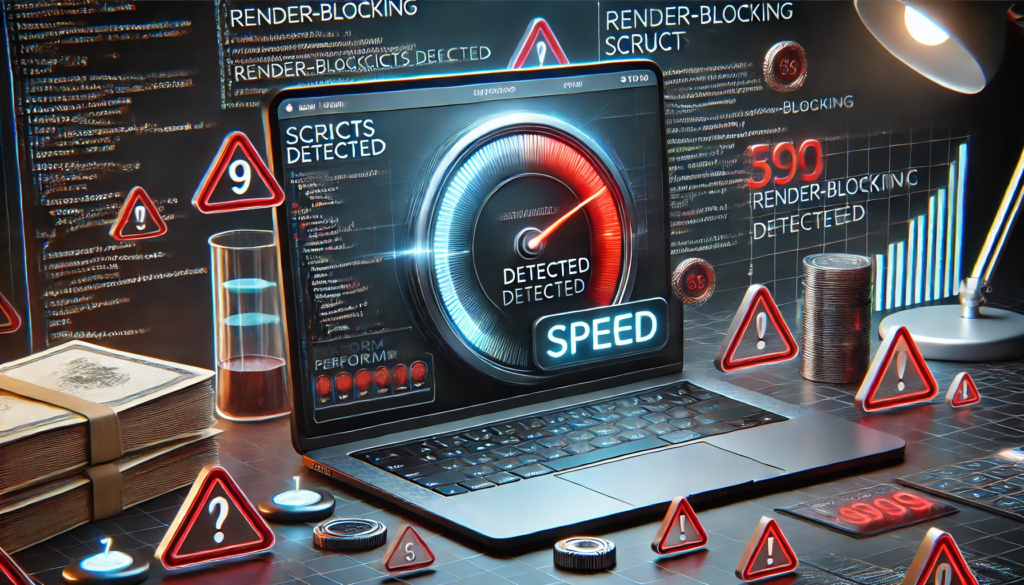
3. Inline Critical JavaScript
For very small, essential scripts, inline them directly into the HTML:
<script>
document.addEventListener("DOMContentLoaded", function() {
console.log("Critical script loaded");
});
</script>
- Reduces extra HTTP requests.
- Best for small scripts that need to execute immediately.
4. Minimize and Compress JavaScript
- Minify JS: Use tools like:
- Terser
- UglifyJS
- Online Minifier: https://www.minifier.org/
- Use Gzip or Brotli compression (enabled via
.htaccess
or server config)<IfModule mod_deflate.c> AddOutputFilterByType DEFLATE application/javascript </IfModule>
5. Load Scripts After User Interaction
Use lazy loading techniques to load scripts when needed:
document.addEventListener("DOMContentLoaded", function() {
let script = document.createElement("script");
script.src = "heavy-script.js";
document.body.appendChild(script);
});
6. Use a CDN for Faster Delivery
- Host scripts on CDNs (Content Delivery Networks) like:
- Cloudflare
- jsDelivr
- Google Hosted Libraries
- Example:
<script src="https://cdn.jsdelivr.net/npm/jquery@3.6.0/dist/jquery.min.js" defer></script>
7. Optimize Third-Party Scripts
- Delay loading ads & tracking scripts (e.g., Google Analytics, Facebook Pixel).
- Use Google Tag Manager (GTM) to manage script execution effectively.
8. Remove Unused JavaScript
- Identify unused JavaScript via Chrome DevTools (
Coverage
tab). - Disable unnecessary plugins or scripts.
9. Optimize WordPress-Specific Render-Blocking
Since you use Yoast SEO & custom SEO setup, consider:
- Perfmatters (Premium plugin for script management)
- WP Rocket (Eliminates render-blocking scripts)
- Asset CleanUp (Disables unnecessary scripts per page)
10. Preload Critical Resources
Preloading key scripts can help:
<link rel="preload" href="important-script.js" as="script">
✅ Final Recommendation:
- Use
defer
andasync
wisely for scripts. - Minify, compress, and use a CDN for JavaScript files.
- Lazy load third-party scripts (ads, analytics).
- Use a caching & optimization plugin in WordPress.
Let me know if you need WordPress-specific tweaks! 🚀
TAGS: #render-blockingscripts, #eliminaterender-blocking, #improvepagespeed, #WordPressperformance