Estimated Reading Time: 2 min
To open images from WordPress posts in a popup box (also known as a lightbox) without using a plugin, you can achieve this using a combination of HTML, CSS, and JavaScript.
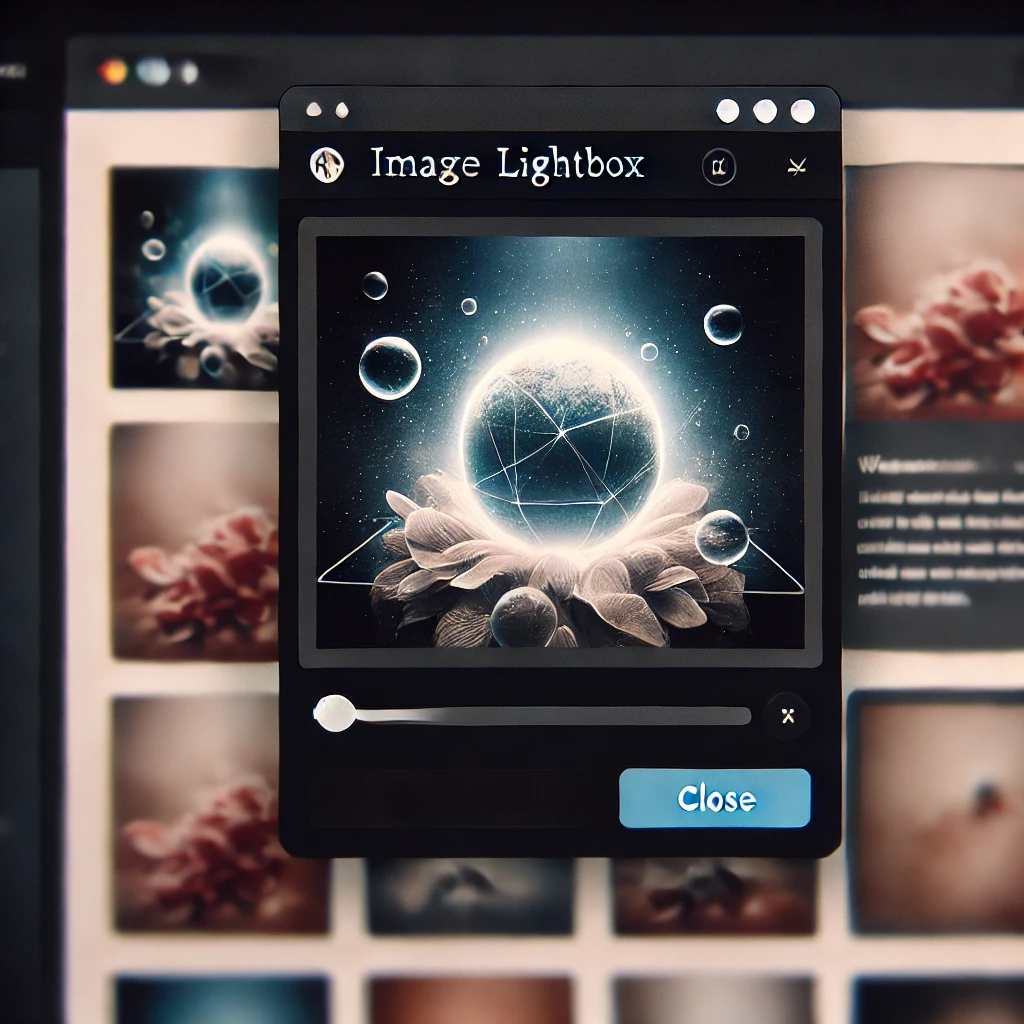
Steps to Implement:
- Modify Your Post Content
- Ensure your images are wrapped inside
<a>
tags with a class, e.g.,.popup-img
.
- Ensure your images are wrapped inside
- Add CSS for the Lightbox
- This will create the popup effect.
- Add JavaScript for Functionality
- JavaScript will handle opening and closing the popup.
1. Modify WordPress Post Content
Ensure your images are wrapped with a link:
<a href="image-url.jpg" class="popup-img">
<img src="image-url.jpg" alt="Image Description" width="300">
</a>
If you’re using the WordPress editor, switch to the HTML view and wrap your images manually.
2. Add CSS (Lightbox Styling)
This will make the Lightbox appear as an overlay:
/* Lightbox Background */
.lightbox {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.8);
justify-content: center;
align-items: center;
}
/* Lightbox Image */
.lightbox img {
max-width: 90%;
max-height: 90%;
box-shadow: 0px 0px 10px white;
border-radius: 10px;
}
/* Close Button */
.lightbox .close {
position: absolute;
top: 20px;
right: 30px;
font-size: 40px;
color: white;
cursor: pointer;
}
3. Add PopUp JavaScript (Functionality)
Add this before the closing </body>
tag in your theme’s footer.php
file or inside a custom JavaScript file:
<script>
document.addEventListener("DOMContentLoaded", function () {
let lightbox = document.createElement("div");
lightbox.className = "lightbox";
lightbox.innerHTML = '<span class="close">×</span><img>';
document.body.appendChild(lightbox);
let lightboxImg = lightbox.querySelector("img");
let closeBtn = lightbox.querySelector(".close");
document.querySelectorAll(".popup-img").forEach(link => {
link.addEventListener("click", function (e) {
e.preventDefault();
lightboxImg.src = this.href;
lightbox.style.display = "flex";
});
});
closeBtn.addEventListener("click", function () {
lightbox.style.display = "none";
});
lightbox.addEventListener("click", function (e) {
if (e.target !== lightboxImg) {
lightbox.style.display = "none";
}
});
});
</script>
4. Implementing PopUp in WordPress
- If using a custom theme, add the CSS to
style.css
and the JavaScript infooter.php
. - If using the block editor (Gutenberg), you can manually wrap images with
<a>
tags inside the HTML block.
Final Outcome
- Clicking an image will open it in a pop up (lightbox).
- Clicking outside the image or on the close button (
×
) will close the box. - Works without any plugin!
Would you like additional enhancements, such as smooth animations? 🚀