Estimated Reading Time: 4 min
To create a Custom 3D Sphere Tag Cloud Plugin for WordPress using CSS and JavaScript, follow these steps:
Steps to Build the Plugin:
- Create the Plugin Folder & File Structure
wp-content/plugins/3d-tag-cloud/
3d-tag-cloud.php
(Main Plugin File)assets/js/tagcloud.js
(JavaScript for 3D Effect)assets/css/style.css
(CSS for Styling)
1. Create the Main Plugin File: 3d-tag-cloud.php
This file registers the plugin, enqueues scripts, and creates a shortcode.
<?php
/**
* Plugin Name: 3D Tag Cloud Sphere
* Description: A custom WordPress plugin to display tags in a 3D sphere using CSS and JavaScript.
* Version: 1.0
* Author: Your Name
* License: GPL2
*/
if (!defined('ABSPATH')) exit; // Exit if accessed directly
// Enqueue Scripts and Styles
function tagcloud_enqueue_scripts() {
wp_enqueue_style('tagcloud-style', plugin_dir_url(__FILE__) . 'assets/css/style.css');
wp_enqueue_script('tagcloud-js', plugin_dir_url(__FILE__) . 'assets/js/tagcloud.js', array('jquery'), null, true);
}
add_action('wp_enqueue_scripts', 'tagcloud_enqueue_scripts');
// Shortcode to Display the Tag Cloud
function tagcloud_shortcode() {
$tags = get_tags(array('orderby' => 'count', 'order' => 'DESC'));
if (empty($tags)) {
return '<p>No tags available.</p>';
}
$output = '<div id="tagcloud-container"><div class="tagcloud-sphere">';
foreach ($tags as $tag) {
$link = get_tag_link($tag->term_id);
$output .= "<a href='{$link}' class='tag-item' data-weight='{$tag->count}'>{$tag->name}</a>";
}
$output .= '</div></div>';
return $output;
}
add_shortcode('tagcloud_sphere', 'tagcloud_shortcode');
?>
2. Create the JavaScript File: assets/js/tagcloud.js
This script generates the 3D spinning tag cloud effect.
document.addEventListener("DOMContentLoaded", function () {
let radius = 150;
let tags = document.querySelectorAll(".tag-item");
let tagElements = [];
let angleX = Math.PI / 200;
let angleY = Math.PI / 200;
// Position Tags in Sphere
function setupSphere() {
let numTags = tags.length;
tags.forEach((tag, i) => {
let k = -1 + (2 * (i + 1) - 1) / numTags;
let phi = Math.acos(k);
let theta = Math.sqrt(numTags * Math.PI) * phi;
let x = radius * Math.cos(theta) * Math.sin(phi);
let y = radius * Math.sin(theta) * Math.sin(phi);
let z = radius * Math.cos(phi);
tag.style.transform = `translate3d(${x}px, ${y}px, ${z}px)`;
tagElements.push({ el: tag, x, y, z });
});
}
function rotateSphere() {
let cosX = Math.cos(angleX);
let sinX = Math.sin(angleX);
let cosY = Math.cos(angleY);
let sinY = Math.sin(angleY);
tagElements.forEach(tag => {
let { x, y, z } = tag;
// Rotation around Y-axis
let tempX = x * cosY - z * sinY;
let tempZ = x * sinY + z * cosY;
x = tempX;
z = tempZ;
// Rotation around X-axis
let tempY = y * cosX - z * sinX;
z = y * sinX + z * cosX;
y = tempY;
tag.x = x;
tag.y = y;
tag.z = z;
let scale = 1.5 + z / radius;
tag.el.style.transform = `translate3d(${x}px, ${y}px, 0) scale(${scale})`;
tag.el.style.opacity = scale / 2;
});
}
function animateSphere() {
rotateSphere();
requestAnimationFrame(animateSphere);
}
setupSphere();
animateSphere();
});
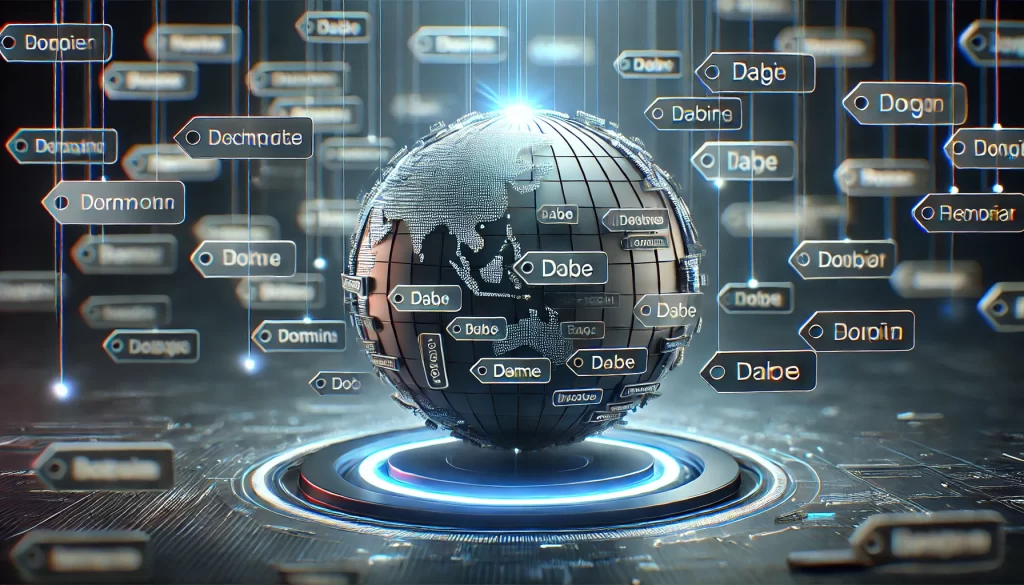
3. Create the CSS File: assets/css/style.css
This styles the 3D tag cloud.
#tagcloud-container {
width: 300px;
height: 300px;
position: relative;
margin: auto;
perspective: 800px;
}
.tagcloud-sphere {
width: 100%;
height: 100%;
position: absolute;
transform-style: preserve-3d;
animation: rotate 10s infinite linear;
}
.tag-item {
position: absolute;
top: 50%;
left: 50%;
font-size: 16px;
font-weight: bold;
text-decoration: none;
color: #333;
transition: transform 0.5s, opacity 0.5s;
}
.tag-item:hover {
color: #0073aa;
transform: scale(1.5);
}
/* Keyframes for Smooth Rotation */
@keyframes rotate {
from { transform: rotateX(0deg) rotateY(0deg); }
to { transform: rotateX(360deg) rotateY(360deg); }
}
4. Activate the Plugin
- Upload the
3d-tag-cloud
folder to thewp-content/plugins/
directory. - Go to WordPress Admin > Plugins and activate 3D Tag Cloud Sphere.
- Add the shortcode
[tagcloud_sphere]
to any post or page.
5. Testing & Customization
- Adjust the radius in
tagcloud.js
for a larger/smaller sphere. - Change CSS styles for different font sizes and animations.
- Modify speed and movement in JavaScript by tweaking
angleX
andangleY
.
Final Result
The plugin will display a 3D rotating sphere with clickable tags, enhancing user engagement.