Estimated Reading Time: 5 min
Building a live trading bot with Python and the Binance API involves several steps. Below is a comprehensive guide to help you get started.
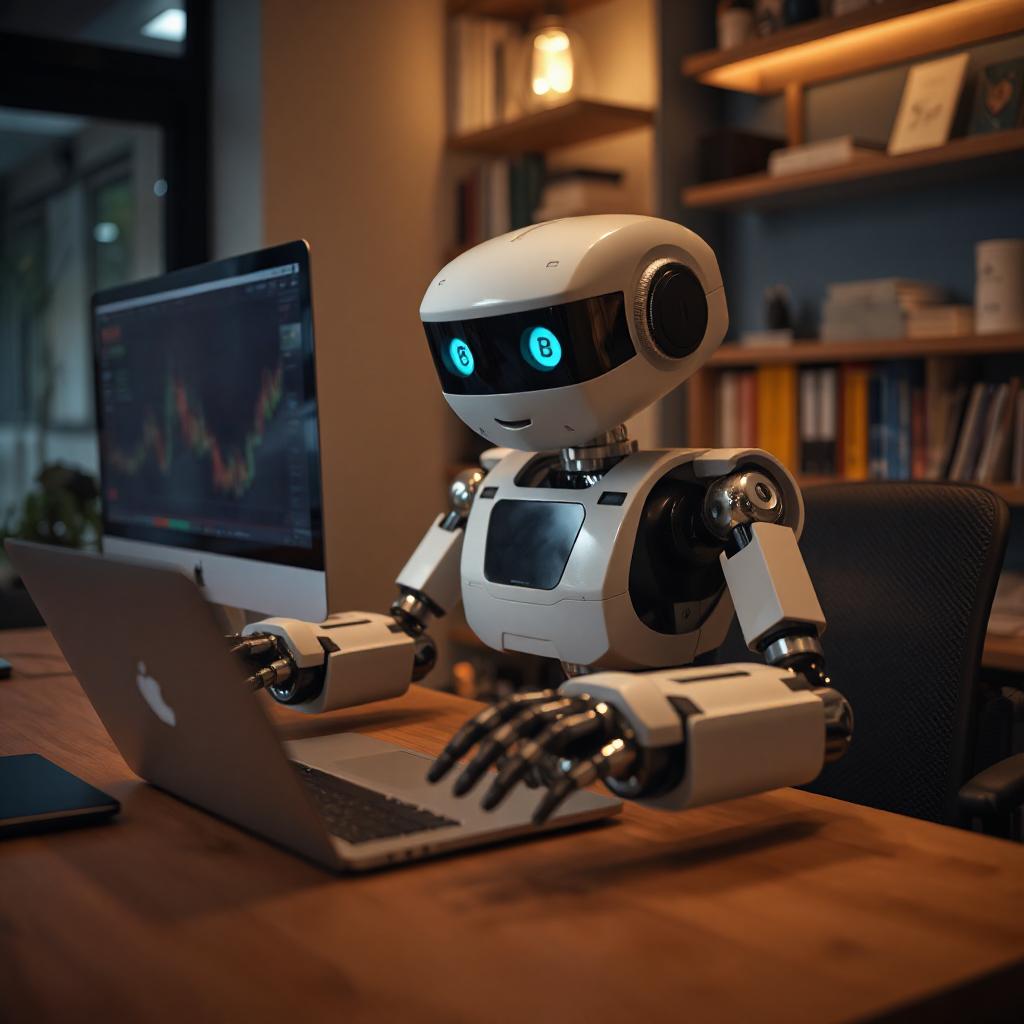
1. Prerequisites
a. Install Required Libraries
You’ll need Python 3.7+ and the following libraries:
pip install python-binance pandas numpy ta
python-binance
: For interacting with the Binance API.pandas
andnumpy
: For data manipulation.ta
(Technical Analysis): For indicators like RSI, MACD, etc.
b. Binance API Key and Secret
- Log in to your Binance account.
- Go to the API Management page.
- Create an API key and secret (enable only necessary permissions, such as trading and account information).
2. Key Components of the Bot
- API Connection: Use
python-binance
to connect to the Binance exchange. - Market Data Fetching: Retrieve live market data for analysis.
- Trading Strategy: Implement a strategy based on technical analysis or rules.
- Order Execution: Place buy/sell orders.
- Risk Management: Incorporate stop-loss, take-profit, and position sizing.
3. Example: Live Trading Bot
Here’s a script that demonstrates how to build a live trading bot:
from binance.client import Client
from binance.enums import *
import pandas as pd
import numpy as np
import time
from ta.momentum import RSIIndicator
# API credentials
API_KEY = 'your_api_key_here'
API_SECRET = 'your_api_secret_here'
# Initialize Binance client
client = Client(API_KEY, API_SECRET)
# Parameters
SYMBOL = 'BTCUSDT'
TRADE_QUANTITY = 0.001 # Amount to trade
RSI_PERIOD = 14 # RSI calculation period
RSI_OVERBOUGHT = 70
RSI_OVERSOLD = 30
def get_historical_data(symbol, interval, lookback):
"""Fetch historical klines (candlestick) data."""
klines = client.get_klines(symbol=symbol, interval=interval, limit=lookback)
data = pd.DataFrame(klines, columns=['time', 'open', 'high', 'low', 'close', 'volume',
'close_time', 'quote_asset_volume', 'trades',
'taker_buy_base', 'taker_buy_quote', 'ignore'])
data['time'] = pd.to_datetime(data['time'], unit='ms')
data['close'] = data['close'].astype(float)
return data[['time', 'close']]
def calculate_rsi(data):
"""Calculate RSI using the ta library."""
rsi = RSIIndicator(data['close'], RSI_PERIOD)
data['rsi'] = rsi.rsi()
return data
def place_order(symbol, side, quantity, order_type=ORDER_TYPE_MARKET):
"""Place a market order."""
try:
order = client.create_order(
symbol=symbol,
side=side,
type=order_type,
quantity=quantity
)
print(f"Order placed: {order}")
except Exception as e:
print(f"Error placing order: {e}")
def trading_strategy():
"""Main trading loop."""
while True:
try:
# Fetch data
data = get_historical_data(SYMBOL, Client.KLINE_INTERVAL_1MINUTE, RSI_PERIOD + 1)
data = calculate_rsi(data)
last_rsi = data['rsi'].iloc[-1]
print(f"Latest RSI: {last_rsi}")
# Trading logic
if last_rsi < RSI_OVERSOLD:
print("RSI below oversold threshold. Buying...")
place_order(SYMBOL, SIDE_BUY, TRADE_QUANTITY)
elif last_rsi > RSI_OVERBOUGHT:
print("RSI above overbought threshold. Selling...")
place_order(SYMBOL, SIDE_SELL, TRADE_QUANTITY)
# Delay to avoid exceeding rate limits
time.sleep(60)
except Exception as e:
print(f"Error: {e}")
time.sleep(60)
if __name__ == "__main__":
trading_strategy()
from binance.client import Client
from binance.enums import *
import pandas as pd
import numpy as np
import time
from ta.momentum import RSIIndicator
# API credentials
API_KEY = 'your_api_key_here'
API_SECRET = 'your_api_secret_here'
# Initialize Binance client
client = Client(API_KEY, API_SECRET)
# Parameters
SYMBOL = 'BTCUSDT'
TRADE_QUANTITY = 0.001 # Amount to trade
RSI_PERIOD = 14 # RSI calculation period
RSI_OVERBOUGHT = 70
RSI_OVERSOLD = 30
def get_historical_data(symbol, interval, lookback):
"""Fetch historical klines (candlestick) data."""
klines = client.get_klines(symbol=symbol, interval=interval, limit=lookback)
data = pd.DataFrame(klines, columns=['time', 'open', 'high', 'low', 'close', 'volume',
'close_time', 'quote_asset_volume', 'trades',
'taker_buy_base', 'taker_buy_quote', 'ignore'])
data['time'] = pd.to_datetime(data['time'], unit='ms')
data['close'] = data['close'].astype(float)
return data[['time', 'close']]
def calculate_rsi(data):
"""Calculate RSI using the ta library."""
rsi = RSIIndicator(data['close'], RSI_PERIOD)
data['rsi'] = rsi.rsi()
return data
def place_order(symbol, side, quantity, order_type=ORDER_TYPE_MARKET):
"""Place a market order."""
try:
order = client.create_order(
symbol=symbol,
side=side,
type=order_type,
quantity=quantity
)
print(f"Order placed: {order}")
except Exception as e:
print(f"Error placing order: {e}")
def trading_strategy():
"""Main trading loop."""
while True:
try:
# Fetch data
data = get_historical_data(SYMBOL, Client.KLINE_INTERVAL_1MINUTE, RSI_PERIOD + 1)
data = calculate_rsi(data)
last_rsi = data['rsi'].iloc[-1]
print(f"Latest RSI: {last_rsi}")
# Trading logic
if last_rsi < RSI_OVERSOLD:
print("RSI below oversold threshold. Buying...")
place_order(SYMBOL, SIDE_BUY, TRADE_QUANTITY)
elif last_rsi > RSI_OVERBOUGHT:
print("RSI above overbought threshold. Selling...")
place_order(SYMBOL, SIDE_SELL, TRADE_QUANTITY)
# Delay to avoid exceeding rate limits
time.sleep(60)
except Exception as e:
print(f"Error: {e}")
time.sleep(60)
if __name__ == "__main__":
trading_strategy()
4. Key Components Explained
a. Market Data Fetching
- The
get_historical_data
function retrieves candlestick data (e.g., open, close prices) from Binance.
b. Technical Analysis
- Uses the
ta
library to calculate the Relative Strength Index (RSI), a popular momentum oscillator.
c. Order Execution
- The
place_order
function sends market orders to Binance. - Replace
ORDER_TYPE_MARKET
withORDER_TYPE_LIMIT
if you want to use limit orders.
d. Risk Management
- Use stop-loss and take-profit levels based on your strategy.
- Position sizing should align with your risk tolerance.
5. Next Steps: Enhancements
- Logging: Use Python’s
logging
module to record bot activity. - Backtesting: Test your strategy on historical data before live trading.
- Advanced Strategies:
- Incorporate more indicators like MACD, Bollinger Bands, etc.
- Use AI/ML models for predictions.
- Error Handling:
- Handle API rate limits and connection issues.
- Implement retries and fail-safes.
- Deployment:
- Run the bot on a cloud server (e.g., AWS, Google Cloud) for 24/7 operation.
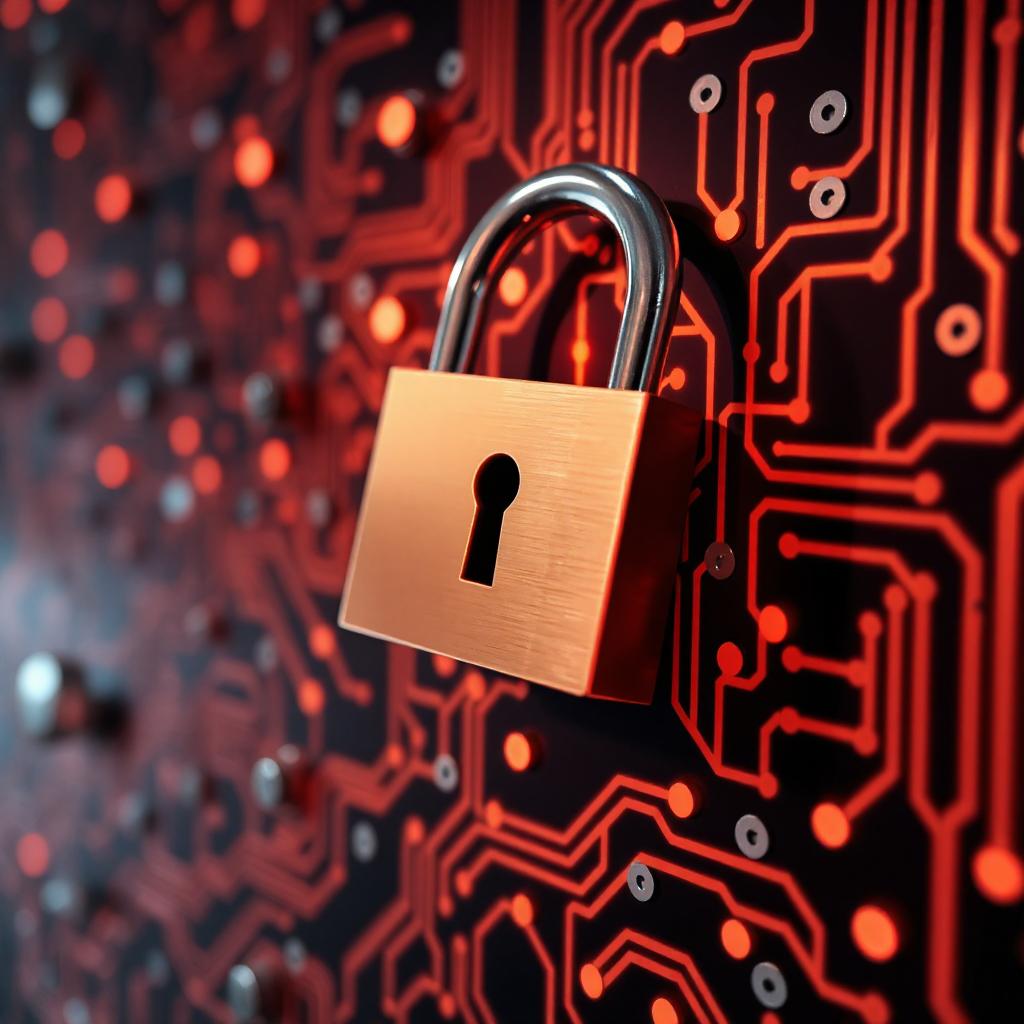
6. Security Best Practices
- Secure API Keys: Store keys in environment variables or a secure secrets manager.
- IP Whitelisting: Restrict API access to specific IP addresses.
- Read/Write Permissions: Enable only necessary permissions for the API key.
Would you like help with any specific part, such as backtesting, deploying on a server, or enhancing the trading strategy?