Estimated Reading Time: 2 min
To collect subscribers in WordPress using PHP, you can create a custom form and handle the submission using a WordPress action. Here’s a step-by-step guide:
1. Create a Subscription Form
Add the following form to your WordPress theme or a custom block/widget:
<form method="POST" action="">
<label for="email">Subscribe to our Newsletter:</label>
<input type="email" id="email" name="subscriber_email" placeholder="Enter your email" required>
<button type="submit" name="submit_subscriber">Subscribe</button>
</form>
2. Handle Form Submission in PHP
Add this code to your theme’s functions.php
file:
function handle_subscriber_submission() {
if (isset($_POST['submit_subscriber']) && isset($_POST['subscriber_email'])) {
$email = sanitize_email($_POST['subscriber_email']);
// Validate email address
if (!is_email($email)) {
wp_die('Invalid email address. Please go back and try again.');
}
// Save email to the database (example with a custom table)
global $wpdb;
$table_name = $wpdb->prefix . 'subscribers'; // Custom table name
// Check if the table exists (if not, create it)
if ($wpdb->get_var("SHOW TABLES LIKE '$table_name'") != $table_name) {
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id mediumint(9) NOT NULL AUTO_INCREMENT,
email varchar(255) NOT NULL,
date datetime DEFAULT CURRENT_TIMESTAMP NOT NULL,
PRIMARY KEY (id),
UNIQUE (email)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
// Insert email into the database
$wpdb->insert(
$table_name,
array(
'email' => $email,
'date' => current_time('mysql')
)
);
// Confirmation message
wp_redirect(home_url('/thank-you')); // Redirect to a thank-you page
exit;
}
}
add_action('init', 'handle_subscriber_submission');
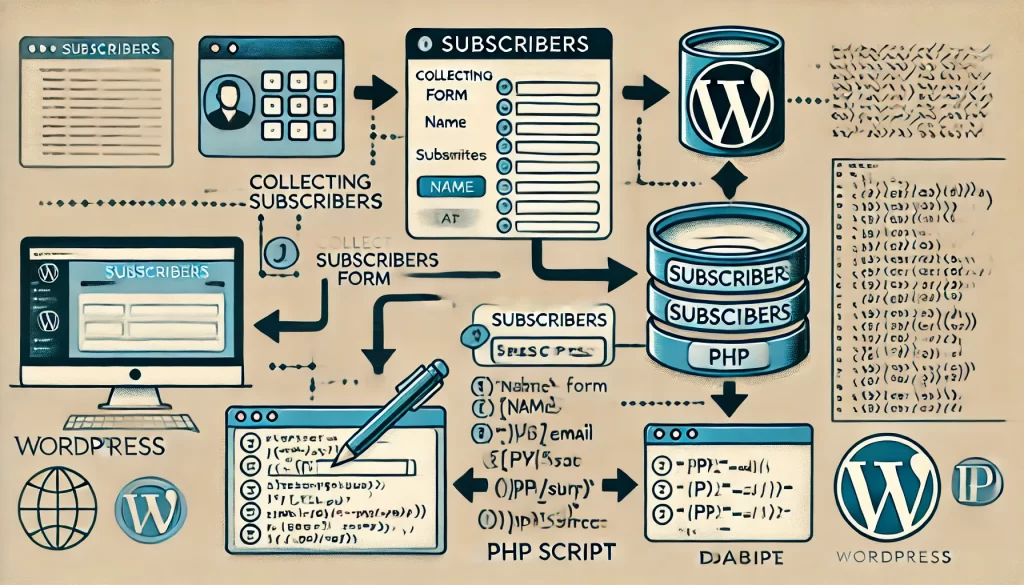
3. Create a Thank-You Page
Create a “Thank You” page in your WordPress admin where users are redirected after subscribing.
4. Access the Collected Emails
You can access the subscriber emails directly in the database under the wp_subscribers
table. Alternatively, you can display them in the admin area using a custom admin page.
Optional Enhancements:
- Send Confirmation Email
Add email functionality usingwp_mail()
to confirm subscription:wp_mail($email, 'Subscription Confirmed', 'Thank you for subscribing!');
- Integrate with Mailchimp or Other Services
Use Mailchimp API or any other service API to sync subscriptions. - Add reCAPTCHA
Integrate Google reCAPTCHA for spam prevention.
Would you like help extending this functionality or customizing it further?